布局与控件的关系:
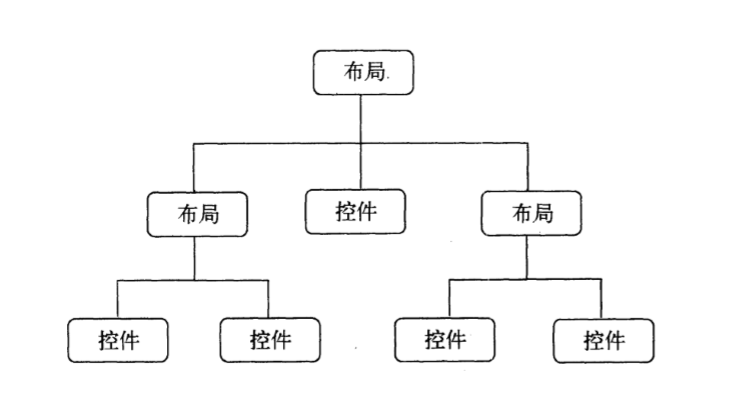
一、线性布局(LinearLayout)
这个布局会将它所包含的控件在线性方向上依次排列。
android:orientation
属性指定控件排列方向:
vertical:垂直方向
horizontal:水平方向
example:
垂直方向: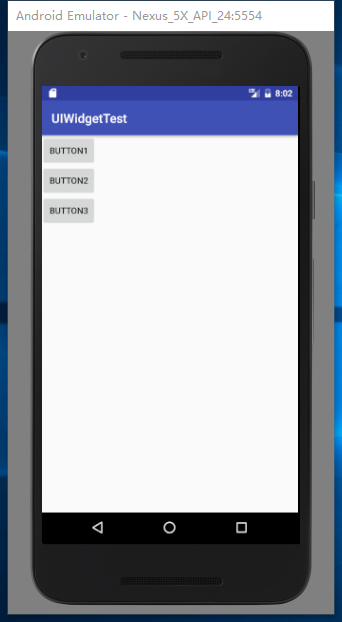
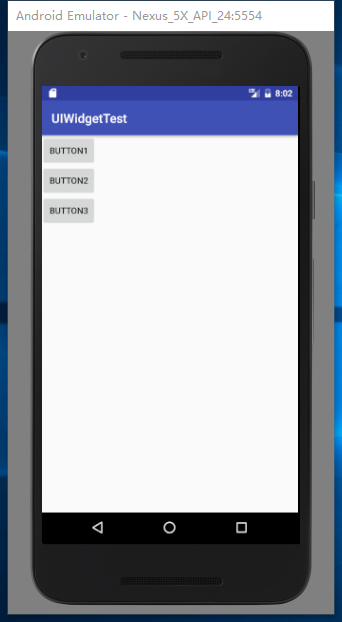
水平方向: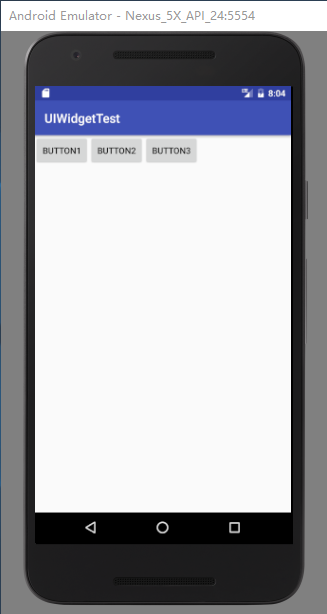
注:当LinearLayout的排列方向是horizontal,内部控件的宽度不能指定为match_parent,因为这样的话单独一个控件就会将整个水平方向占满;同理, 当LinearLayout的排列方向是vertical,内部控件的高度不能指定为match_parent
android:layout_gravity
属性用来指定文字在控件中的对齐方式:
注:当LinearLayout的排列方向是horizontal,只有垂直方向上的对齐方式才会生效, 因为水平方向上的长度不固定,每加一个控件,水平方向的长度都会改变,无法指定该方向上的对齐方式,同理,当LinearLayout的排列方向是vertical, 只有水平方向上的对齐方式才会生效
android:layout_weight
属性允许使用比例方式指定控件的大小
example:
1 2 3 4 5 6 7 8 9 10 11 12
| <EditText android:id="@+id/input_message" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:hint="Type something"/> <Button android:id="@+id/send" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:text="Send"/>
|
虽然<EditText>
和<Button>
的width值都为0dp,但是此时的宽度由weight属性来决定,<EditText>
和<Button>
的weight属性都为1,表示EditText和Button将在水平方向平分宽度。若EditText的值为a,Button的值为b,则EditText所占的长度为总长度的a/(a+b),Button的长度为b/(a+b)。
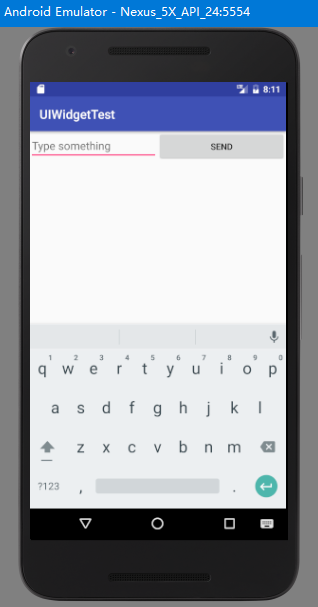
二、相对布局(RelativeLayout)
通过相对定位的方式让控件出现在布局的任何位置
1. 相对于父布局进行定位
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_alignParentTop="true" android:text="button1"/> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_alignParentTop="true" android:text="button2"/> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:text="button3"/> <Button android:id="@+id/button4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_alignParentLeft="true" android:text="button4"/> <Button android:id="@+id/button5" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_alignParentRight="true" android:text="button5"/>
</RelativeLayout>
|
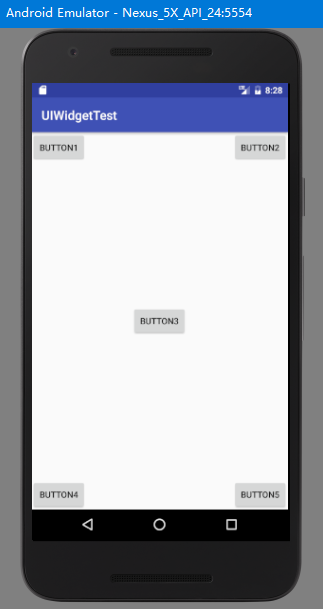
2. 相对于控件进行定位
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_above="@+id/button3" android:layout_toLeftOf="@id/button3" android:text="button1"/> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_above="@id/button3" android:layout_toRightOf="@id/button3" android:text="button2"/> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:text="button3"/> <Button android:id="@+id/button4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/button3" android:layout_toLeftOf="@id/button3" android:text="button4"/> <Button android:id="@+id/button5" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/button3" android:layout_toRightOf="@id/button3" android:text="button5"/> </RelativeLayout>
|

三、帧布局(FrameLayout)
所有的控件默认摆放在布局的左上角
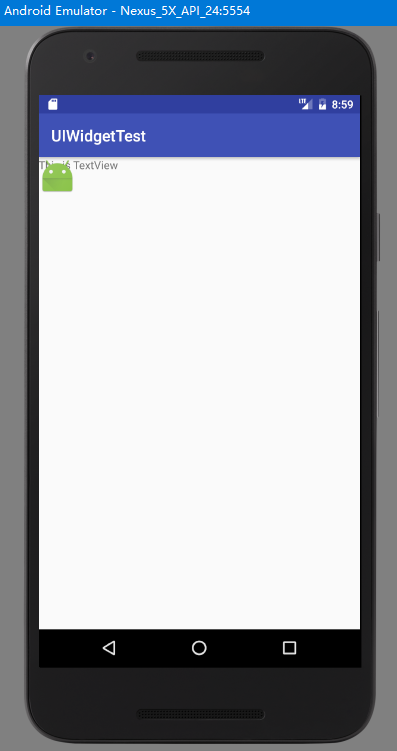
上图中图片与文字重叠
四、百分比布局
a. PercentFrameLayout
b. PercentRelativeLayout
- 在build.gradle中添加百分比布局库的依赖
1 2 3 4 5 6 7
| dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) androidTestCompile('com.android.support.test.espresso:espresso-core:2.2.2', {exclude group: 'com.android.support', module: 'support-annotations'}) compile 'com.android.support:appcompat-v7:24.2.1' + compile 'com.android.support:percent:24.2.1' testCompile 'junit:junit:4.12' }
|
- 修改activity_main.xml中的代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <android.support.percent.PercentFrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/button1" android:text="Button1" android:layout_gravity="left|top" app:layout_widthPercent="50%" app:layout_heightPercent="50%"/> <Button android:id="@+id/button2" android:text="Button2" android:layout_gravity="right|top" app:layout_widthPercent="50%" app:layout_heightPercent="50%"/> <Button android:id="@+id/button3" android:text="Button3" android:layout_gravity="left|bottom" app:layout_widthPercent="50%" app:layout_heightPercent="50%"/> <Button android:id="@+id/button4" android:text="Button4" android:layout_gravity="right|bottom" app:layout_widthPercent="50%" app:layout_heightPercent="50%"/> </android.support.percent.PercentFrameLayout>
|
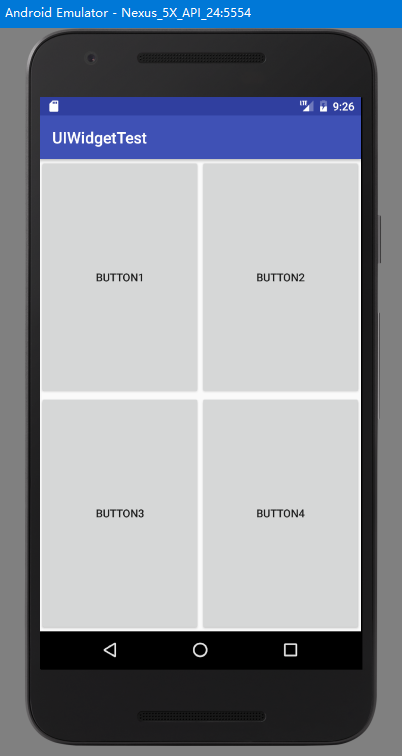